- Published on
Getting Started with Git
3 min read
- Authors
- Name
- Christopher Morales
- @
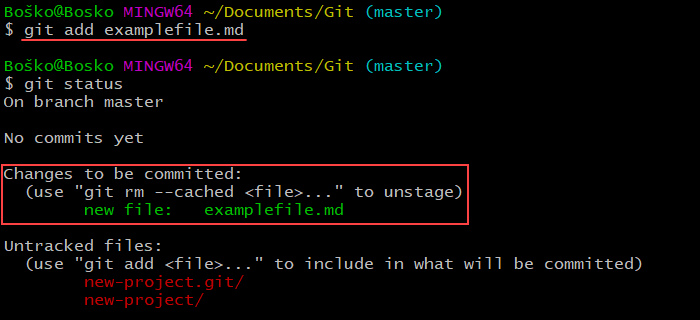
Table of Contents
- Short Summary
- Process
- Creating/initializing Git Repository
- Configure Push Defaults
- Adding Files to Staging Area
- Include .gitignore in Staging
- Check Staging Status
- Configure User Information
- Configure Credential Helper (Optional)
- Commit Changes
- Add a Remote Repository
- Rename the Default Branch (Optional)
- Push Changes to GitHub
- Enter GitHub Credentials (if asked)
- Managing Nested Git Repository: Submodules vs Ignoring Directories
- Approach 1: Using Git Submodules
- Remove Nested Directories from Current Tracking
- Add as Submodules
Short Summary
This article provides a comprehensive, step-by-step guide on initializing a Git repository, configuring essential settings, and connecting to a remote repository on GitHub. Whether you're a beginner or looking for a quick reference, this guide will walk you through the process with clear instructions and explanations.
Process
Creating/initializing Git Repository
This command initializes a new Git repository in the current directory:
git init
Configure Push Defaults
Set the default behavior for 'git push' to be more straightforward:
git config --global push.default simple
Adding Files to Staging Area
Add all files to the staging area. The asterisk (*) represents all files:
git add *
Include .gitignore in Staging
Ensure that your .gitignore file is included in the staging area to specify files and directories to ignore during version control:
git add .gitignore
Check Staging Status
View the status of your changes in the staging area:
git status
Configure User Information
Set your email and username to associate your commits with your GitHub account:
git config --global user.email "youremail@umich.edu"
git config --global user.name "Your X. Name"
Configure Credential Helper (Optional)
Optional but convenient steps to cache your credentials for a specified period:
git config --global credential.helper cache
git config --global credential.helper 'cache --timeout=604800'
Commit Changes
Commit the changes with a descriptive message:
git commit -m "first commit"
Add a Remote Repository
Link your local repository to a remote repository on GitHub:
git remote add origin URL_LINK
Rename the Default Branch (Optional)
Optionally, rename the default branch from "master" to "main":
git branch -m master main
Push Changes to GitHub
Push your committed changes to the remote repository on GitHub:
git push -u origin main
Enter GitHub Credentials (if asked)
Enter your GitHub username and password when prompted
Managing Nested Git Repository: Submodules vs Ignoring Directories
When working with Git, one of the challenges developers might face is how to handle nested Git repositories within a main project. This situation typically arises when you've inadvertently initialized a Git repository within another Git repository. This can cause issues, as seen when trying to commit changes and encountering errors due to these nested directories being treated as untracked files.
Let's explore two practical approaches to managing these nested Git repositories: using Git submodules and ignoring the directories.
Approach 1: Using Git Submodules
Git submodules allow you to keep a reference to specific commits in other repositories, rather than including all their files directly in your parent repository. This method is ideal if these nested directories are indeed separate projects that you want to maintain independently.
Here’s how to set up submodules:
Remove Nested Directories from Current Tracking
First, you'll want to stop tracking these directories in your main project:
git rm --cached sub-repository/file-path -r
For example:
git rm --cached Project/Neural_Network_Deep_Learning_Project -r
git rm --cached Project/Website_Project_2.0 -r
Add as Submodules
Next, add these as submodules by using their remote repository URLs:
git submodule add <repository-url> sub-repository/file-path
For example:
git submodule add <repository-url> Project/Neural_Network_Deep_Learning_Project
git submodule add <repository-url> Project/Website_Project_2.0